Using Real-world Images(사람이랑 말그림 구분해보기)
1. 내가 대~충 이해한것
-이제까지는 어느정도 전처리된 이미지만을 이용하여 학습해왔다.
-예를들면, 사진은 거의 정중앙에 있고, 정형화된 사이즈의 것들이었음.
-근데 실제로는 안그렇잖아?
-사물은 측면이나 움직임에 따라 같은것이어도 바뀌고, 사진 정중앙에 항상 있으라는 법도, 그것이 모두 같은 사이즈라는 법도없음.
2. 실제 사진을 학습시키기 위해서
-전처리 과정이 필요하다(이미지의 사이즈를 통일시키는등)
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# All images will be rescaled by 1./255
train_datagen = ImageDataGenerator(rescale=1/255)
# Flow training images in batches of 128 using train_datagen generator
train_generator = train_datagen.flow_from_directory(
'/tmp/horse-or-human/', # This is the source directory for training images
target_size=(300, 300), # All images will be resized to 150x150
batch_size=128,
# Since we use binary_crossentropy loss, we need binary labels
class_mode='binary')
-모델링
model = tf.keras.models.Sequential([
# Note the input shape is the desired size of the image 300x300 with 3 bytes color
# This is the first convolution
tf.keras.layers.Conv2D(16, (3,3), activation='relu', input_shape=(300, 300, 3)),
tf.keras.layers.MaxPooling2D(2, 2),
# The second convolution
tf.keras.layers.Conv2D(32, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
# The third convolution
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
# The fourth convolution
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
# The fifth convolution
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
# Flatten the results to feed into a DNN
tf.keras.layers.Flatten(),
# 512 neuron hidden layer
tf.keras.layers.Dense(512, activation='relu'),
# Only 1 output neuron. It will contain a value from 0-1 where 0 for 1 class ('horses') and 1 for the other ('humans')
tf.keras.layers.Dense(1, activation='sigmoid')
])
주석에 있는 내용처럼, 이번엔 말이냐 사람이냐를 수치로 표현하기 때문에 바이너리와 효율이 좋은 패러미터가 사용되었다. 같은 이유에서 컴파일시에도 조금 다르다.
from tensorflow.keras.optimizers import RMSprop
model.compile(loss='binary_crossentropy',
optimizer=RMSprop(lr=0.001),
metrics=['accuracy'])
-학습
history = model.fit(
train_generator,
steps_per_epoch=8,
epochs=15,
verbose=1)
-예측
classes = model.predict(images, batch_size=10)
print(classes[0])
프린트 값이 1이면 사람이고 0이면 말이다.
3. 더 정확한 학습을 위하여(Adding automatic validation to test accuracy)
-학습셋뿐만아니라 검증셋(validation set)을 이용하여 학습하면 좀더 정확한 예측을 할 수 있다.
-전처리에서 검증셋에 대한 generator를 추가한다
-학습(validation에 대한 항목이 추가되어있다)
history = model.fit(
train_generator,
steps_per_epoch=8,
epochs=15,
verbose=1,
validation_data = validation_generator,
validation_steps=8)
-이뿐만아니라 overfitting의 문제, 이미지 사이즈에 따른 영향등, 학습에 영향을 미치는 요소에 대해서 생각해 볼 필요가 있다고 말한다. 맨 처음에 나왔던 overfitting에 대해서는 여기 퀴즈에서 또 물어보고 있으므로 이번엔 외부자료를 찾아 읽어보았다. https://untitledtblog.tistory.com/68
[머신러닝] - Overfitting (과적합)
1. 개요 머신 러닝 (machine learning)에서 overfitting은 학습데이터를 과하게 잘 학습하는 것을 뜻한다. 일반적으로 학습 데이터는 실제 데이터의 부분집합인 경우가 대부분이다. 따라서, 아래의 그래
untitledtblog.tistory.com
4. submit
기다리고 기다린 서브밋의 시간...
문제는 아래와 같습니다.
Below is code with a link to a happy or sad dataset which contains 80 images, 40 happy and 40 sad. Create a convolutional neural network that trains to 100% accuracy on these images, which cancels training upon hitting training accuracy of >.999
Hint -- it will work best with 3 convolutional layers.
이제 한 몇번하다보니 비슷~비슷~
콜백함수로 0.999를 넘으면 epoch를 종료시키고, 합성곱 레이어는 3개쓰면 된다고 한다.
마지막 이고하니 전체를 남겨볼까한다.
def train_happy_sad_model():
# Please write your code only where you are indicated.
# please do not remove # model fitting inline comments.
DESIRED_ACCURACY = 0.999
class myCallback(tf.keras.callbacks.Callback):
# Your Code
def on_epoch_end(self, epoch, logs={}):
if(logs.get('acc') > DESIRED_ACCURACY):
print("\nReached 99.9% accuracy so cancelling training!")
self.model.stop_training = True
callbacks = myCallback()
# This Code Block should Define and Compile the Model. Please assume the images are 150 X 150 in your implementation.
model = tf.keras.models.Sequential([
# Your Code Here
tf.keras.layers.Conv2D(16, (3,3), activation='relu', input_shape=(150, 150, 3)),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(32, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(512, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
from tensorflow.keras.optimizers import RMSprop
model.compile(loss='binary_crossentropy', optimizer=RMSprop(lr=0.001), metrics=['accuracy'])
# This code block should create an instance of an ImageDataGenerator called train_datagen
# And a train_generator by calling train_datagen.flow_from_directory
from tensorflow.keras.preprocessing.image import ImageDataGenerator
train_datagen = ImageDataGenerator(rescale=1/255)
# Please use a target_size of 150 X 150.
train_generator = train_datagen.flow_from_directory(
# Your Code Here
'/tmp/h-or-s/',
target_size=(150, 150),
batch_size=128,
class_mode='binary')
# Expected output: 'Found 80 images belonging to 2 classes'
# This code block should call model.fit_generator and train for
# a number of epochs.
# model fitting
history = model.fit_generator(
# Your Code Here
train_generator,
steps_per_epoch=8,
epochs=15,
verbose=1,
callbacks=[callbacks]
)
# model fitting
return history.history['acc'][-1]
디버깅은 항상그렇지만; 아주 사소한 것들에서 오류를 내고 말았다.
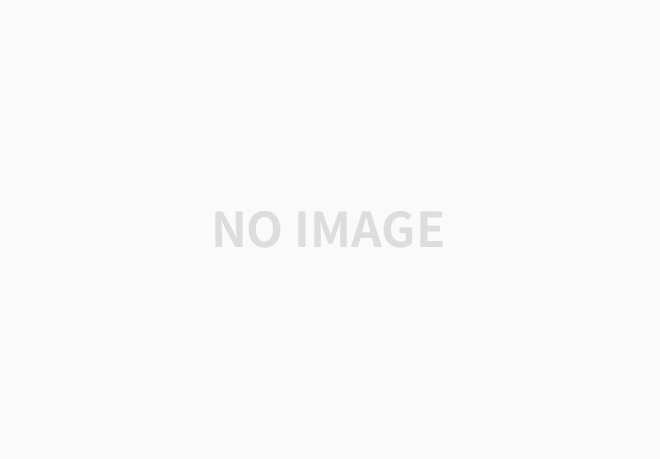
input_shape와 train_generator의 사이즈가 안맞아서 혼남
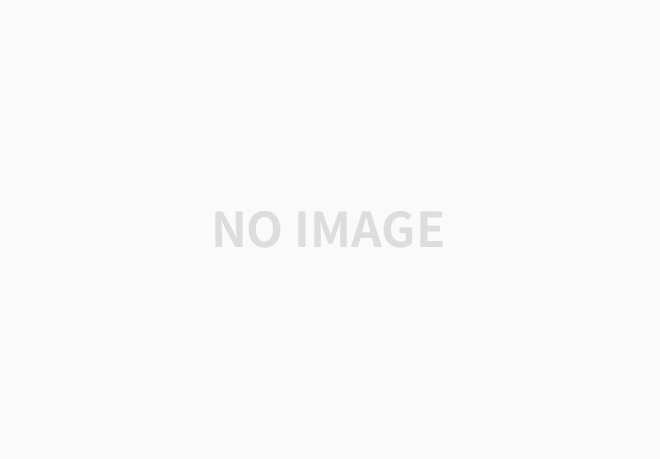
콜백쓰루~ 왜 콜백이 안먹지;;
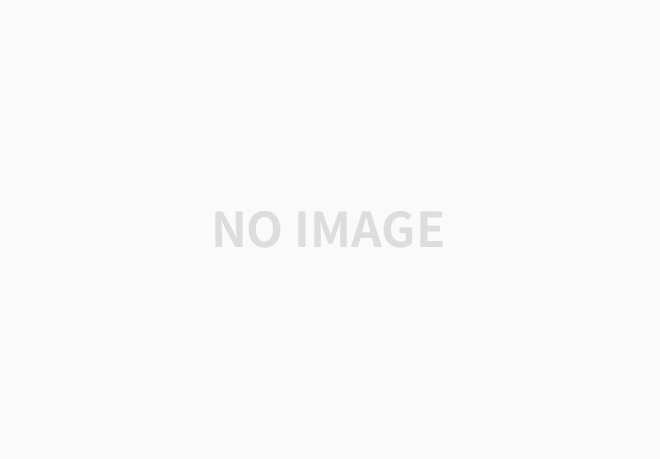
그거야 콜백쓴다고 안써놨었으니까~허허...
5. 수료
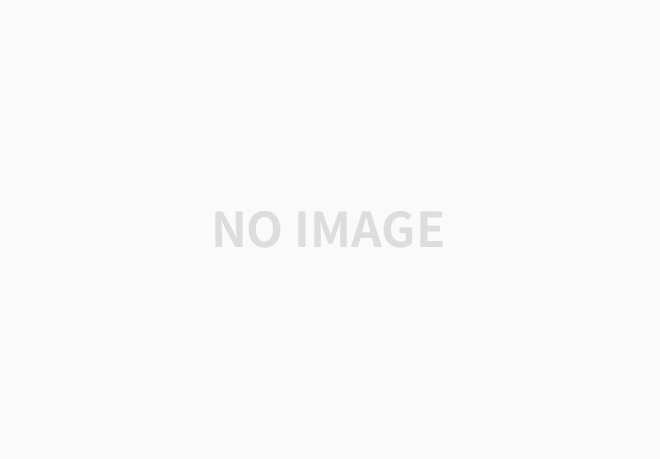
헤헷.. 계정에서 실명입력하면 수료증을 준다.
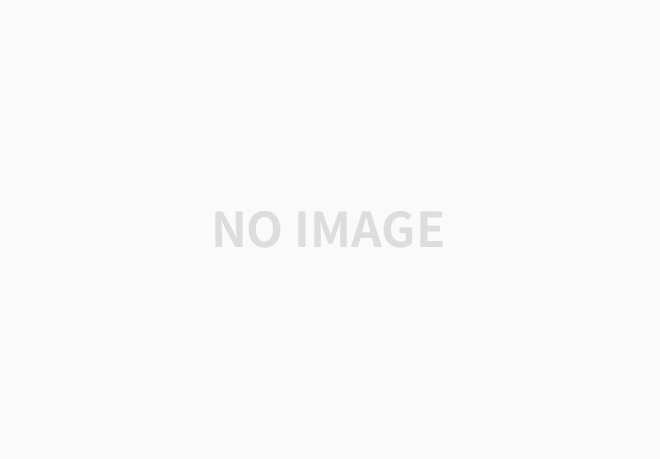
성적은 오픈북이었으니까 의미없긴하지만서도...나는 코딩보단 저 퀴즈가 더 긴장됬다.
일정시간안에 3번인가 밖에 재시험이 불가능해서리...다행히 모두 2번안에는 끝났다.
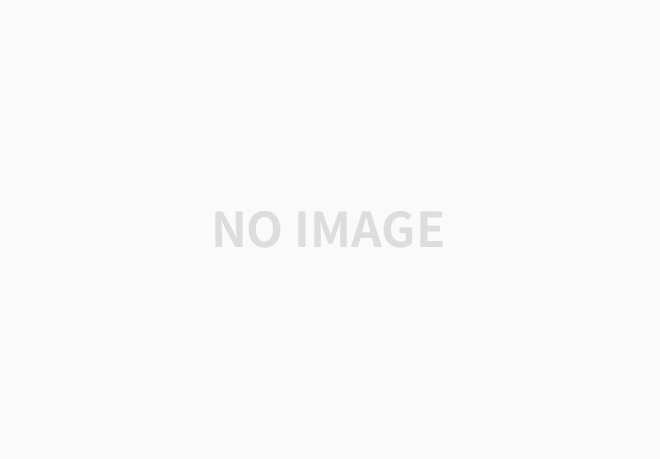
수료증은 이런식으로 나오고 PDF로 다운하거나, 링크드인에 공유할 수 있다.
전체적으로는 TensorFlow 입문하시려는 분께 굉장히 재미있는 강좌고,
특히 이미지처리에 대해서 관심이 있는 분은 정말 좋은 강좌가 될것같다.
이 다음은 텐서플로 자격증을 노려봐야 하겠으나, 찾아본 바로는
시험내용은 이 강좌안의 내용이 거의 그대로 나오고,
시험내용보다는 환경준비에 빡치시는 분들이 많이 계신듯하다..
100불을 내고 기념적으로 가지고있어야 하는건가...
머신러닝쪽 자격증이 많지는 않으니 있으면 재미있을꺼 같기도하고...
작년에 구글에서 머신러닝 부트캠프를 했는데 거기서는 약간 지원등이 있었다고 들었다.
또 그런거 안하나...
어쨌든 다음주 월요일부턴 GCP니까 텐서플로우는 찬찬히 생각해 보려고한다.
'ML' 카테고리의 다른 글
TensorFlow 도전기 (with Coursera 3일차) (0) | 2021.06.05 |
---|---|
TensorFlow 도전기 (with Coursera 2일차) (0) | 2021.06.04 |
TensorFlow 도전기 (with Coursera 1일차) (0) | 2021.06.04 |
댓글